- ORDER BY
SELECT * 또는 칼럼명
FROM 테이블명
WHERE 조건절
ORDER BY 칼럼명 DESC
- 내림차순 DESC
- 오름차순 ASC(디폴트이기 때문에 쓸 일이 별로 없음)
- ORDER BY는 데이터베이스에 저장된 데이터 자체의 순서를 바꾸진 않는다
Q1: products 테이블에서 가격이 20 이상인 물건들 중에 가장 비싼 것부터 정렬해라
SELECT *
FROM Products
WHERE price >= 20
ORDER BY price DESC
Q2: products 테이블에서 가격이 가장 비싼 물건 1개만 출력해라
SELECT *
FROM Products
ORDER BY price DESC
LIMIT 1
- 결과의 개수를 제한하는 LIMIT 활용
- '가격이 비싼 물건 상위 n개를 출력해라' 한다면 LIMIT의 개수를 n으로 바꿔주면됌
Q3: products 테이블에서 가격이 가장 싼 물건 1개만 출력해라
SELECT *
FROM Products
ORDER BY price ASC
LIMIT 1
- 오름차순인 ASC는 디폴트이기 때문에 자주 사용하진 않지만, 가장 작은 값을 불러올 때 활용할 수 있다
- 마찬가지로 '가격이 싼 물건 하위 n개를 출력해라' 한다면 LIMIT의 개수를 n으로 바꿔주면됌
- HackerRank
1. https://www.hackerrank.com/challenges/name-of-employees/problem?isFullScreen=true
Employee Names | HackerRank
Print employee names.
www.hackerrank.com
Q: Write a query that prints a list of employee names (i.e.: the name attribute) from the Employee table in alphabetical order.
Input Format
The Employee table containing employee data for a company is described as follows:
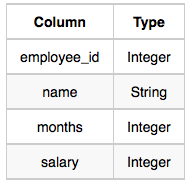
A:
SELECT name
FROM employee
ORDER BY name
Employee Salaries | HackerRank
Print the names of employees who earn more than $2000 per month and have worked at the company for less than 10 months.
www.hackerrank.com
Q: Write a query that prints a list of employee names (i.e.: the name attribute) for employees in Employee having a salary greater than $2000 per month who have been employees for less than 10 months.
Sort your result by ascending employee_id.
Input Format
The Employee table containing employee data for a company is described as follows:
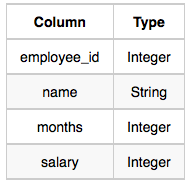
A:
SELECT name
FROM employee
WHERE salary > 2000 AND months < 10
ORDER BY employee_id
- MySQL 문자열 자르기
- LEFT(칼럼명 또는 문자열, 문자열 길이)
SELECT LEFT('20140323', 4)
=> 2014
- RIGHT(칼럼명 또는 문자열, 문자열 길이)
SELECT RIGHT('20140323', 4)
=> 0323
- SUBSTRING(칼럼명 또는 문자열, 시작 위치, 길이) = SUBSTR( )
SELECT SUBSTR('20140323', 1, 4)
=> 2014
SELECT SUBSTR('20140323', 5)
=> 0323
-- 길이가 생략됐을 땐, 5번째부터 끝까지
3. https://www.hackerrank.com/challenges/more-than-75-marks/problem?isFullScreen=true
Higher Than 75 Marks | HackerRank
Query the names of students scoring higher than 75 Marks. Sort the output by the LAST three characters of each name.
www.hackerrank.com
Q: Query the Name of any student in STUDENTS who scored higher than 75 Marks.
Order your output by the last three characters of each name.
If two or more students both have names ending in the same last three characters (i.e.: Bobby, Robby, etc.), secondary sort them by ascending ID.
Input Format
The STUDENTS table is described as follows:
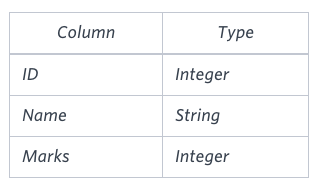
- 마지막 세글자를 기준으로 정렬하긴 하는데, 그 세글자가 똑같은 학생들은 id를 오름차순 기준으로 정렬해라
A:
SELECT name
FROM students
WHERE marks > 75
ORDER BY RIGHT(name, 3), id
- 두번째 기준은 ,(콤마)찍고 써주기
+) 한 줄 주석 처리는 --
한꺼번에 주석 처리는 /* ... */
- MySQL 소수점 처리
- CEIL( ) 올림
SELECT CEIL(5.5)
=> 6
- FLOOR( ) 내림
SELECT FLOOR(5.5)
=> 5
- ROUND( ) 반올림
SELECT ROUND(5.556901, 4)
=> 5.5569
4. https://www.hackerrank.com/challenges/weather-observation-station-15/problem?isFullScreen=true
Weather Observation Station 15 | HackerRank
Query the Western Longitude for the largest Northern Latitude under 137.2345, rounded to 4 decimal places.
www.hackerrank.com
Q: Query the Western Longitude (LONG_W) for the largest Northern Latitude (LAT_N) in STATION that is less than 137.2345.
Round your answer to 4 decimal places.
Input Format
The STATION table is described as follows:
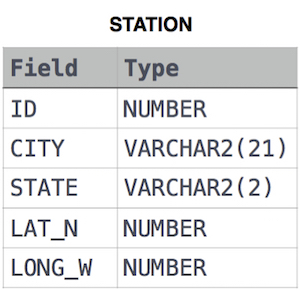
A:
SELECT ROUND(long_w, 4)
FROM station
WHERE lat_n < 137.2345
ORDER BY lat_n DESC
LIMIT 1
- 출력해야 하는건 LONG_W이니까 SELECT 다음에 바로 ROUND를 써주기, 4번째자리까지
- Largest Northern Latitude라고 했으니까 137.2345보다 작은 LAT_N 중에서 제일 큰 한 개를 출력
→ LAT_N을 내림차순(큰 순으로)으로 정렬한 후 1개로 제한하기
이 문제 어렵다.. 복습 필수!
'SQL' 카테고리의 다른 글
Aggregate Function 집계 함수 - COUNT/SUM/AVG/MIN/MAX (0) | 2023.07.07 |
---|---|
MySQL 정규표현식 REGEXP + 해커랭크 예제 풀이 (0) | 2023.06.29 |
HackerRank 해커랭크 SQL 예제 추가 풀이 (1) (0) | 2023.06.23 |
WHERE문 해커랭크 예제 풀이(DISTINCT, NOT LIKE) (0) | 2023.06.22 |
LIKE/IN/BETWEEN/IS NULL (0) | 2023.06.20 |
- ORDER BY
SELECT * 또는 칼럼명
FROM 테이블명
WHERE 조건절
ORDER BY 칼럼명 DESC
- 내림차순 DESC
- 오름차순 ASC(디폴트이기 때문에 쓸 일이 별로 없음)
- ORDER BY는 데이터베이스에 저장된 데이터 자체의 순서를 바꾸진 않는다
Q1: products 테이블에서 가격이 20 이상인 물건들 중에 가장 비싼 것부터 정렬해라
SELECT *
FROM Products
WHERE price >= 20
ORDER BY price DESC
Q2: products 테이블에서 가격이 가장 비싼 물건 1개만 출력해라
SELECT *
FROM Products
ORDER BY price DESC
LIMIT 1
- 결과의 개수를 제한하는 LIMIT 활용
- '가격이 비싼 물건 상위 n개를 출력해라' 한다면 LIMIT의 개수를 n으로 바꿔주면됌
Q3: products 테이블에서 가격이 가장 싼 물건 1개만 출력해라
SELECT *
FROM Products
ORDER BY price ASC
LIMIT 1
- 오름차순인 ASC는 디폴트이기 때문에 자주 사용하진 않지만, 가장 작은 값을 불러올 때 활용할 수 있다
- 마찬가지로 '가격이 싼 물건 하위 n개를 출력해라' 한다면 LIMIT의 개수를 n으로 바꿔주면됌
- HackerRank
1. https://www.hackerrank.com/challenges/name-of-employees/problem?isFullScreen=true
Employee Names | HackerRank
Print employee names.
www.hackerrank.com
Q: Write a query that prints a list of employee names (i.e.: the name attribute) from the Employee table in alphabetical order.
Input Format
The Employee table containing employee data for a company is described as follows:
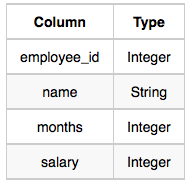
A:
SELECT name
FROM employee
ORDER BY name
Employee Salaries | HackerRank
Print the names of employees who earn more than $2000 per month and have worked at the company for less than 10 months.
www.hackerrank.com
Q: Write a query that prints a list of employee names (i.e.: the name attribute) for employees in Employee having a salary greater than $2000 per month who have been employees for less than 10 months.
Sort your result by ascending employee_id.
Input Format
The Employee table containing employee data for a company is described as follows:
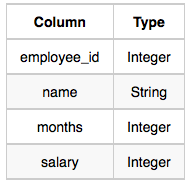
A:
SELECT name
FROM employee
WHERE salary > 2000 AND months < 10
ORDER BY employee_id
- MySQL 문자열 자르기
- LEFT(칼럼명 또는 문자열, 문자열 길이)
SELECT LEFT('20140323', 4)
=> 2014
- RIGHT(칼럼명 또는 문자열, 문자열 길이)
SELECT RIGHT('20140323', 4)
=> 0323
- SUBSTRING(칼럼명 또는 문자열, 시작 위치, 길이) = SUBSTR( )
SELECT SUBSTR('20140323', 1, 4)
=> 2014
SELECT SUBSTR('20140323', 5)
=> 0323
-- 길이가 생략됐을 땐, 5번째부터 끝까지
3. https://www.hackerrank.com/challenges/more-than-75-marks/problem?isFullScreen=true
Higher Than 75 Marks | HackerRank
Query the names of students scoring higher than 75 Marks. Sort the output by the LAST three characters of each name.
www.hackerrank.com
Q: Query the Name of any student in STUDENTS who scored higher than 75 Marks.
Order your output by the last three characters of each name.
If two or more students both have names ending in the same last three characters (i.e.: Bobby, Robby, etc.), secondary sort them by ascending ID.
Input Format
The STUDENTS table is described as follows:
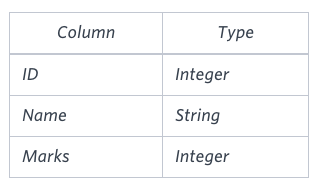
- 마지막 세글자를 기준으로 정렬하긴 하는데, 그 세글자가 똑같은 학생들은 id를 오름차순 기준으로 정렬해라
A:
SELECT name
FROM students
WHERE marks > 75
ORDER BY RIGHT(name, 3), id
- 두번째 기준은 ,(콤마)찍고 써주기
+) 한 줄 주석 처리는 --
한꺼번에 주석 처리는 /* ... */
- MySQL 소수점 처리
- CEIL( ) 올림
SELECT CEIL(5.5)
=> 6
- FLOOR( ) 내림
SELECT FLOOR(5.5)
=> 5
- ROUND( ) 반올림
SELECT ROUND(5.556901, 4)
=> 5.5569
4. https://www.hackerrank.com/challenges/weather-observation-station-15/problem?isFullScreen=true
Weather Observation Station 15 | HackerRank
Query the Western Longitude for the largest Northern Latitude under 137.2345, rounded to 4 decimal places.
www.hackerrank.com
Q: Query the Western Longitude (LONG_W) for the largest Northern Latitude (LAT_N) in STATION that is less than 137.2345.
Round your answer to 4 decimal places.
Input Format
The STATION table is described as follows:
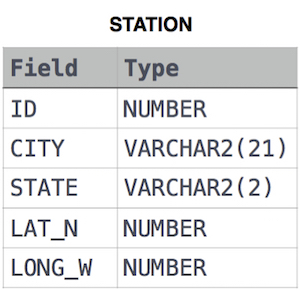
A:
SELECT ROUND(long_w, 4)
FROM station
WHERE lat_n < 137.2345
ORDER BY lat_n DESC
LIMIT 1
- 출력해야 하는건 LONG_W이니까 SELECT 다음에 바로 ROUND를 써주기, 4번째자리까지
- Largest Northern Latitude라고 했으니까 137.2345보다 작은 LAT_N 중에서 제일 큰 한 개를 출력
→ LAT_N을 내림차순(큰 순으로)으로 정렬한 후 1개로 제한하기
이 문제 어렵다.. 복습 필수!
'SQL' 카테고리의 다른 글
Aggregate Function 집계 함수 - COUNT/SUM/AVG/MIN/MAX (0) | 2023.07.07 |
---|---|
MySQL 정규표현식 REGEXP + 해커랭크 예제 풀이 (0) | 2023.06.29 |
HackerRank 해커랭크 SQL 예제 추가 풀이 (1) (0) | 2023.06.23 |
WHERE문 해커랭크 예제 풀이(DISTINCT, NOT LIKE) (0) | 2023.06.22 |
LIKE/IN/BETWEEN/IS NULL (0) | 2023.06.20 |